Automatic Reference Counting (ARC) does:
1.Memory management, job of the compiler.
2.Never need to type retain or release again and again.
3.Reducing crashes and memory leaks.
4.Releases each object the instant, if it is no longer used.
5.Apps run as fast as ever, with predictable, smooth performance.
Before ARC, we had to manually retain/release/autorelease objects to ensure they would “stick around” for as long as you needed them. Forgetting to send retain to an object, or releasing it too many times would cause your app to leak memory or crash.
In Xcode 4.2, in addition to syntax checking as you type, the new Apple LLVM compiler makes it possible to offload the burden of manual memory management to the compiler, introspecting your code to decide when to release objects.
Apple’s documentation describes ARC as follows:
“Automatic Reference Counting (ARC) is a compiler-level feature that simplifies the process of managing object lifetimes (memory management) in Cocoa applications.”
Before ARC, we had to write:
NSObject *obj = [[NSObject alloc] init];
// do some stuff
[obj release];
—
Or if we are using autorelease then we had to write:
-(NSObject*) someMethod {
NSObject *obj = [[[NSObject alloc] init] autorelease];
return obj; // will be deallocated by autorelease pool later
}
But with ARC enabled we have to write only :
NSObject *obj = [[NSObject alloc] init];
// do some stuff
The ARC pre-compilation step will auto-magically turn it into this:
NSObject *obj = [[NSObject alloc] init];
// do some stuff
[obj release]; // **Added by ARC**
How Automatic Reference Counting Works
ARC is a pre-compilation step that adds retain/release/autorelease statements to your code for you.
This is not a Garbage Collection, and reference counted memory has not disappeared, it has simply been automated. It may sound like a bit of an after thought, but considering how many features in Objective-C are implemented by pre-processing source files before compiling, ARC is really par for the course.
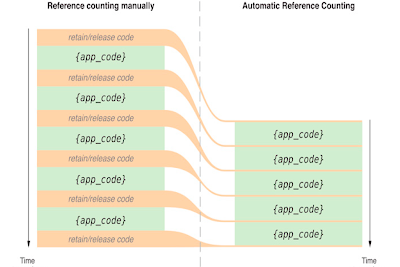
Enabling ARC in Your Project
To enable ARC simply set the Objective-C Automatic Reference Counting option in your Xcode project’s Build Settings to YES. Behind the scenes this sets the -fobjc-arc compiler flag that enables ARC.
—
Including Code that is not ARC Compliant
—According to Apple’s documentation: “ARC interoperates with manual reference counting code on a per-file basis. If you want to continue using manual reference counting for some files, you can do so.”
—This means some files can use ARC and some files can be spared from it’s magical grasp. Here are the steps for bulk excluding files from ARC at compile time. At the time of writing, many popular libraries are not ARC ready, to get around this follow these steps:
—Click on your Project in the Xcode project tree
—Click on the Target
—Select the Build Phases tab
—Expand the Compile Sources section
—Select one or more files you want to exclude from ARC
—Press the return key
—Type -fno-objc-arc
—Press the return key again
—Each file selected now has a -fno-objc-arc compiler flag set and will be excluded from ARC
Migrating Existing Projects to ARC
Xcode 4.2 provides a conversion tool that migrates existing code to ARC, and helps you manually convert code that cannot be automatically migrated.
1. Open your non-ARC compliant project and go to Edit > Refactor > Convert to Objective-C ARC.
—
No comments:
Post a Comment